Beginners Guide to Arithmetic Operations on Korn Shell Variables
Place an integer expression in two pairs of parentheses ((…)) to invoke an Arithmetic evaluation. For readability, place a space before and after any arithmetic operator within the double pair of parentheses. The table below lists the standard arithmetic operations.
Operator | Operation | Example | Result |
---|---|---|---|
+ | Addition | ((x = 24 + 25)) | 49 |
- | Subtraction | ((x = 100 - 25)) | 75 |
* | Multiplication | ((x = 4 * 5)) | 20 |
/ | Division | ((x = 10 / 3)) | 3 |
% | Integer remainder | ((x = 10 % 3)) | 1 |
All arithmetic operations are performed using integer arithmetic, which can cause minor surprises:
$ x=15.38
$ y=15.72
$ ((z = x + y))
$ echo $z
30
Everything is integer arithmetic. Note what happens when division is performed in a calculation. For example, when computing the percentage of the eligible voters who actually voted, the following statement would most likely assign 0 to prct:
((prct = voted / eligible_voters * 100))
The following algebraically equivalent statement is the correct way to compute the percentage of eligible voters in the Korn shell:
(((prct = voted * 100 / eligible_voters))
Arithmetic Precedence
- Expressions (operations) within parentheses are evaluated first. Use a single pair of parentheses around an expression to force it to be evaluated first.
- Multiplication (*) and division (% and /) have greater precedence than addition (+) and subtraction (-).
- Arithmetic expressions are evaluated from left to right.
- When there is the slightest doubt, use parentheses to force the evaluation order.
Bit-wise Operations
The six bit-wise operators are listed in the table below:
Operator | Operation | Example | Result |
---|---|---|---|
# | base | 2#1101010 or 16#6A | 10#106 |
<< | Shift bits left | ((x = 2#11 << 3)) | 2#11000 |
» | Shift bits right | ((x = 2#1001 » 2)) | 2#10 |
& | Bit-wise AND | ((x = 2#101 & 2#110)) | 2#100 |
Bit-wise OR | ((x = 2#101 | ||
^ | Bit-wise exclusive OR | ((x = 2#101 ^ 2#110)) | 2#11 |
The # operator designates the base of the value that follows it. For example, 2#101 means that the value 101 is a base 2 (binary) value (2#101 would be 5 in the decimal base).
The << operator performs a binary shift left by as many bits as are indicated by the number that follows the operator. The expression 2#10 << 1 yields the value 2#100. The expression 2#10100<< 2 yields the value 2#1010000.
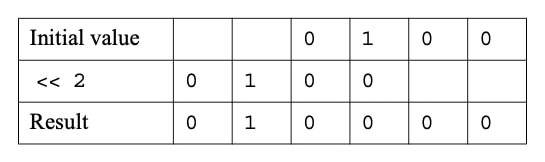
Vacated positions are padded with 0 or 1 based on whether the number is positive or negative, respectively. The » operator performs a binary shift right by as many bits as are indicated by the number that follows the operator. The expression 2#10 » 1 yields the value 2#1. The expression 2#10100 » 2 yields the value 2#101.
The & operator ANDs two binary numbers together. This means that the AND operation is performed on the corresponding digit of 0 or 1 in each number, resulting in a 1 if both digits are 1; otherwise, the result is 0.
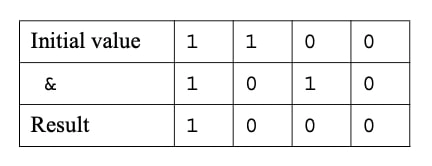
The | operator ORs two binary numbers together. This means that the OR operation is performed on the corresponding digit of 0 or 1 in each number, resulting in a 1 if either digit is a 1; otherwise, the result is 0.
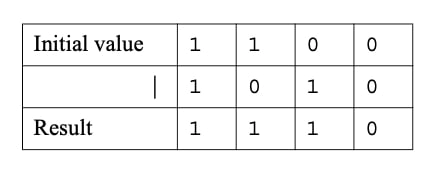
The ^ operator performs an exclusive OR on two binary numbers together. This means that an exclusive OR is performed on the corresponding digit of 0 or 1 in each number, resulting in 1 if only one of the digits is a 1. If both digits are 0 or both are 1, then the result is 0.
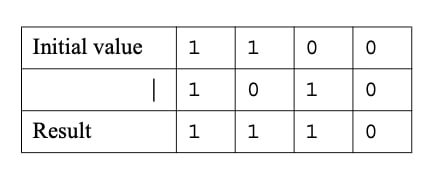