How to Use Comments in Java
Single-line Comments
Comments help easy understanding of code. A single-line comment is used to document the functionality of a single line of code. The code below shows the Java program documented with single-line comments.
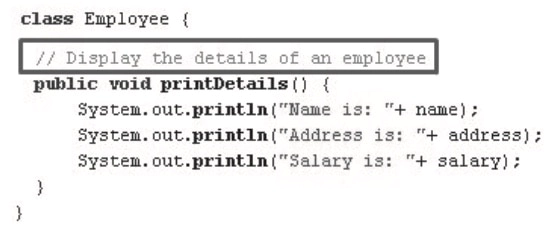
There are two ways of using single-line comments: 1. Beginning-of-line comment - This type of comment can be placed before the code (on a different line). 2. End-of-line comment - This type of comment is placed at the end of the code (on the same line).
Conventions for using single-line comments are as follows:
- Provide a space after the forward slashes.
- Capitalize the first letter of the first word.
The following is the syntax for applying the comments.
Syntax:
// Comment text
The following code shows the different ways of using single-line comments in the Java program.
Code Snippet:
// Declaring a variable
int a = 32;
int b = 64; // Declaring a variable
Multi-line Comments
A multi-line comment is a comment that spans multiple lines. A multi-line comment starts with a forward slash and an asterisk (/*). It ends with an asterisk and a forward slash (*/). Anything that appears between these delimiters is considered to be a comment. The code below shows the Java program documented with multi-line comments.
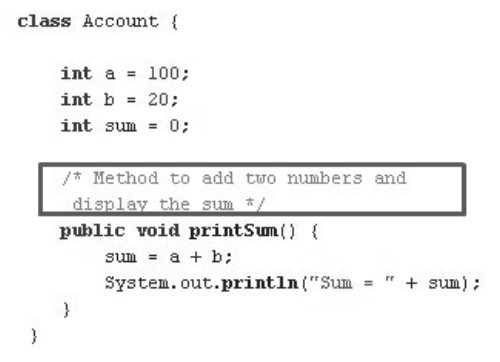
The following code shows a Java program using multi-line comments.
Code Snippet:
/*
This code performs mathematical
operation of adding two numbers.
*/
int a = 20;
int b = 30;
int c;
c = a + b;
Javadoc Comments
A Javadoc comment is used to document public or protected classes, attributes, and methods. It starts /** and ends with */. Everything between the delimiters is a comment, even if it spans multiple lines. The javadoc command can be used for generating Javadoc comments. The code below shows the Java program with Javadoc comment.
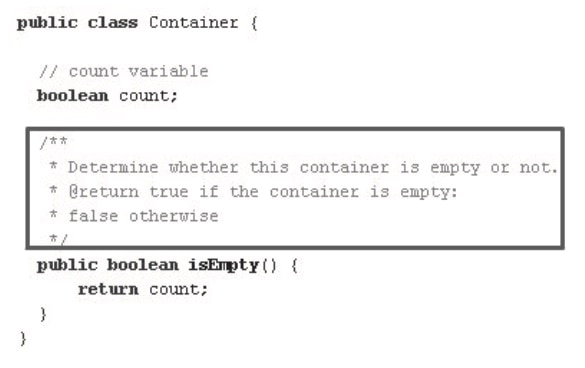
The following code shows the Javadoc comment specified on the method in the Java program.
Code Snippet:
/**
* This program prints a welcome message
* using the println statement.
*/
System.out.println(“Welcome to Java Programming”);