C Pointers and Functions
Pointers and functions get on very well. Whenever data is to be passed to a function, especially large amounts of them, it is easier to pass a pointer to a function and to get back a pointer as the return value of the function.
Now, consider the very simple problem of interchanging two integer values. Suppose A is storing 5 and B is storing 10, after exchanging A should hold 10 and B should hold 5 obviously another temporary location, temp, is used to hold one of the values, while interchanging is taking place.
More on pointers and functions
void exchange (pint1, pint2)
int *pint1, *pint2;
{
int temp;
temp = *pint1;
*pint1 = *pint2;
*pint2 = temp;
}
main()
{
int i1 = -5, i2 = 66, *p1 = &i1, *p2 = &i2;
printf(“il = %d, i2 = %d\n”,i1,i2);
exchange(p1,p2);
printf(“i1 = %d”, i2 = %d\n”, i1,i2);
exchange(&i1, &i2);
printf(“i1 = %d, i2 = %d\n”,i1,i2);
}
OUTPUT:
i1 = -5, i2 = 66
i1 = 66, i2 = -5
i1=-5, i2 = 66
Now consider a more complicated program. The function here searches for a particular value in a linked list. If it is found, it returns a pointer to the node holding the value. Otherwise, it returns a null pointer.
struct entry
{
int value;
struct entry *next;
};
struct entry *find_entry (1pointer, match_value)
struct entry *lpointer;
int match_value;
{
while (lpointer !=(struct entry *) 0)
if (lpointer->value = = match_value)
return (lpointer);
else
lpointer = lpointer->next;
return (struct entry *) 0);
}
main()
{
struct entry n1,n2,n3;
struct entry *lptr, *list_start = &n1;
int i,search;
n1.value = 100;
n1.next = &n2;
n2.value = 200;
n2.next = &n3;
n3.value = 300;
n3.next = (struct entry *) 0;
printf(“ Enter value to locate:”);
scanf(“%d”, &search);
if(lptr != (struct entry *) 0)
printf(“Found %d\n”,lptr->value);
else
printf(“Not found.\n”);
}
OUTPUT:
Enter Value to locate: 200
Found 200.
Enter Value to locate: 400 (Re-run)
Not found 200.
Enter Value to locate: 300 (Re-run)
Found 300.
In fact, the concept of pointers can keep expanding to any extent, you can have an array of pointers. For example, in a class, there are 30 students. You have stored their names. While the no. of students is constant at 30, the no. of characters in their names is variable. Then the simplest thing is to create an array of pointers of size 30, each pointing to a linked list of one name.
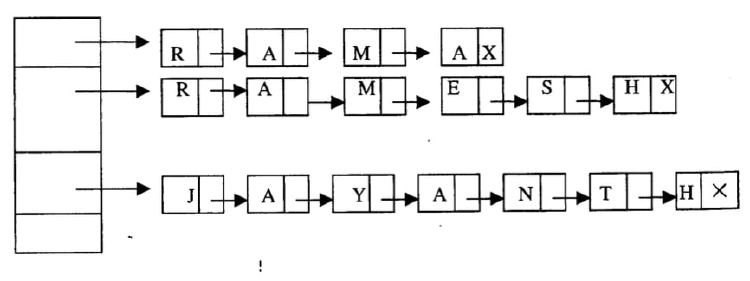