C Pointers - Definition and operation pointers & structures
Pointers are one of the very powerful & special features of C. It simply means instead of using a variable name, you use a pointer ( or indicator) to it. At a simple level, it can be thought of as an address to the variable.
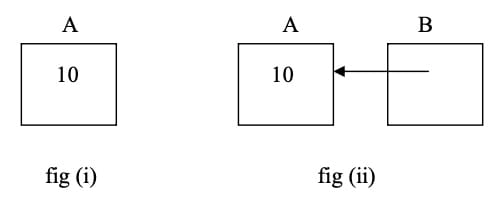
The above figure illustrates the feature. In variable A, the value 10 is stored. Now to access this, you can ask for variable A. On the other hand, you can ask for a pointer like B, shown in fig(ii). If you say B is of the pointer type, then you are actually not interested in the contents of B (which indicates where A is there), but using them you come back to A.
It is like, say, if you are interested in going to a place, you can either know where that place is (normal variables) or know a person who in them knows where the place is situated (pointer variables). Some others also call this " indirection “. You are approaching A indirectly, through its pointer B.
At this stage, one question is likely to arise. Why should we go about this roundabout way, instead of directly going to the variable? One advantage is as in the case of single variables, it is easier to work with normal, direct variable names. But, when you are using large chunks of data, it is easier to work with pointers. Like, say when you have 100 items in an almirah, it is easier to handle a key to the almirah ( a pointer ) rather than the individual items. if you want to hand over these items to someone, it is easier to hand over the key, rather than each of the individual contents. Such situations arise when we are passing parameters to a function and back. It is easier to pass the pointers to the function, than each of those individual items.
These are other advantages also, which become evident as we processed through this chapter. Before that, we see the general format of the pointer variable.
Suppose, we define a variable A as 10 (say)
We say int a=10;
Now, we have to define one more variable, which is a pointer to A, say B (see the fig above). To distinguish that A is an actual variable and B is a pointer, we indicate B as int *B
The asterisk * indicates that B is of the type pointer. Now to finally indicate that B points to A, we write
B= &a
The situation can be depicted as below:
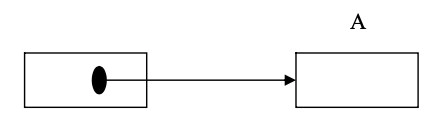
Both A and B are locations. A contains an integer value 10, whereas B contains a pointer to A.
We go back to the steps again: i) Declare A as a variable ii) Declare B as a pointer iii) Link A and B
Now, how do we get the contents of A ( and say store it in X)? The direct way is to say X=A. Alternatively, we can say
X = *B
Then the control goes to B, takes its contents as a pointer to A then goes to A and gets the contents. Now let us illustrate these concepts with a simple program.
Program to illustrate pointers
main()
{
int count = 10,x;
int *int_pointer;
int_pointer = &count;
x = *int_pointer;
printf (“count = %d, x= %d\n”, count,x);
}
OUTPUT:
Count = 10, x = 10
Pointers need not always point to integers only. See the following program.
Further examples of pointers
main()
{
char c = ‘ Q ‘;
char *char_pointer = &c;
printf(“%c %c\n”, c,*char_pointer);
c = ‘/’;
printf(“%c %c\n”, c, *char_pointer);
*char_pointer = ‘(‘;
printf(“%c %c\n”, c,*char_pointer);
}
OUTPUT:
Q Q
/ /
( (
More on pointers
main()
{
int il,i2;
int *p1, *p2;
il = 5;
p1 = &il;
i2 = *p1 / 2 + 10;
p2 = p1;
printf(“il=%d, i2=%d, p1=%d, p2=%d\n”, il,i2,p1,p2);
}
OUTPUT:
i1 = 5, i2 = 12, *p1 = 5, *p2 = 5
These concepts are extremely simple but are very useful and you have to be fully confident of their usage.
Pointers can be used just like normal variables for arithmetic operations for example if you want to add 10 to a value pointed to by prt1 (where ptr1 is a pointer variable), we simply write
*ptr1 = *ptr1 + 10
( Compare: If we want to add 10 to A, we say A=A+10). Similarly we can say *ptr3 = *ptr1 + *ptr2. But we cannot say ptr3=ptr1+ptr2 ( why ?).
Pointers to arrays
When an array of say 100 elements is declared, the compiler creates a storage of 100 consecutive locations, say starting form 1000. If A[ 0 ] = 1000, a[I]=1000+1;A[2]= 1000 +2 etc.. i.e the addresses of the first element a[ 0] is called the base address, to which the location of the element which is required ( 1,2 ….. ) ( which is called the offset is added to get the actual address. We can as well pass a pointer to the base address of the array.
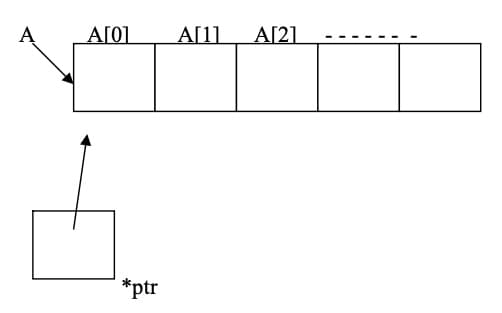
If ptr is the pointer to A, then we can declare
Ptr = & A, which means ptr is a pointer to A. See the following program:
Function to sum the elements of an integer array
int array_sum(array,n)
int array[];
int n;
{
int sum = 0, *ptr;
int *array_end = array + n;
for ( ptr = array; ptr < array_end; ++ptr)
sum += *ptr;
return (sum);
}
main()
{
static int values[10] = { 3, 7, -9, 3, 6, -1, 7, 9, 1, -5};
printf(“The sum is %d\n”,array_sum(values, 10));
}
OUTPUT:
The sum is 21
Just to become familiar with the various operations using strings, we write down a few programs that necessarily operate on strings.
Program to copy a string from one string to another
void copy_string (from,to)
char from,to;
{
for( ; *from != ‘\0’; ++from, ++to)
*to = *from;
*to = ‘\0’;
}
main()
{
static char string1[] = “A string to be copied,”;
static char string2[50];
copy_string(string1, string2);
printf(“%s\n”,string2);
copy_string(“So is this.”,string2);
printf(“%s\n”,string2);
}
OUTPUT:
A string to be copied
So is this.
Write a program to find the number of characters in a string using pointers.
NUMBER OF CHARACTERS IN A GIVEN STRING USING POINTERS
#include<stdio.h>
main()
{
char a[80];
int n;
printf("\n ENTER A STRING :\n");
gets(a);
n=str-len(&a);
printf("\n NUMBER OF CHARACTERS IN THE STRING:%d",n);
}
/* END OF MAIN */
/*FUNCTION str-len*/
str_len(s)
char *s;
{
int i=0;
while (*s!='\0')
{
i++;
s++;
}
retrun(i);
}
/*END OF FUNCTION srt_len*/
OUTPUT:
ENTER A STRING :
Hi! How are you and how are your studies going on ?
OUTPUT:
NUMBER OF CHARACTERS IN THE STRING : 50
Write a program to count number of vowels, consonants, digits, blank-space characters and other characters in a line of text entered form the keyboard.
PROGRAM TO READ TEXT AND TEXT CHARACTERS USING POINTERS
#include<stdio.h>
main()
{
char line[50],s;
int v=0, c=0, d=0, ws=0, other=0;
void scan-line();
printf("\n Enter a line of text:\n");
scanf("%[^\n]",line);
scan-line(line,&v,&c,&d,&ws,&other);
printf("\n Number of Vowels:%d",v);
printf("\n Number of Consonants:%d",c);
printf("\n Number of Digits:%d",d);
printf("\n Number of Blank spaces:%d",ws);
printf("\n Number of other characters:%d",other);
}
/* END OF MAIN */
/*FUNCTION scan_line */
void scan_line (line,pv,pc,pd,pws,pother)
char line[ ];
int pv,pc,*pd,*pws,*pother;
{
char s;
int count=0;
while((s=toupper(line[count])) !='\0')
{
if(s= ='A' || s= ='E' || s= ='I' || s= ='O' || s= ='U')
++*pv;
else if (s>='A'&& S<='Z')
++*pc;
else if (s>='0' && s<'9')
++*pd;
else if (s>==' ' || s= ='\t')
++*pws;
else
++*pother;
++count;
}
}
/* END OF FUNCTION scan_line*/
OUTPUT:
Enter a line of text:
This 1 line is to test all characters in a line !@#$%.
Numbers of Vowels : 14
Number of Consonants:22
Number of digits: 1
Number of Blank spaces : 11
number of other characters : 5
Write a program to copy the contents of one string to another string using pointers.
COPYING A STRING USING POINTERS
#include<stdio.h>
main()
{
char a[80], b[80];
printf("\n ENTER STRING 'A':\n");
gets(a);
printf("\n");
strcopy(&a,&b);
printf("CONTENTS OF STRING 'B' :\N");
output(&b);
}
/*FUNCTION MAIN*/
strcopy(s,d)
char *d,*s;
{
while (*s!='\O')
{
*d = *s;
d++;
s++;
}
*d++ = '\O');
}
/* END OF FUNCTION strcopy */
/*FUNCTION output */
output(s)
char *s;
{
while(*s!='\O')
{
printf("%c",*s);
s++;
}
}
/* END OF FUNCTION output */
OUTPUT:
String A will be copied into String B
CONTENTS OF STRING 'B':
String A will be copied into String B
Write a program to concatenate two strings into a new string.
TO CONCATENATE THE GIVEN TWO STRINGS
#include<stdio.h>
main()
{
char a[80],b[80],c[160];
printf("\n ENTER A STRING :\n");
gets(a);
printf("\n ENTER ANOTHER STRING:\n);
gets(b);
stringcat(&a,&b,&c);
printf("\n THE CONCATENATED STRING IS :\n");
output(&c);
}
/*END OF MAIN */
/* FUNCTION stringcat */
stringcat(s1,s2,d)
char s1,s2,*d;
{
while(*s1 !='\O')
{
*d = *s1;
d++;
s1++;
}
*d++ = ' ';
while (*s2!='\O')
{
*d = *s2;
d++;
s2++;
}
*d ='\O';
}
/*END OF FUNCTION stringcat */
/*FUNCTION output */
output(s)
char *s;
{
while (*s!='\O')
{
printf("%c",*s);
s++;
}
}
/* END OF FUNCTION output */
OUTPUT:
ENTER A STRING: MAHANTESH
ENTER ANOTHER STRING: TEGGI
THE CONCATENATED STRING IS: MAHANTESHTEGGI
Pointers and structures: Pointers can also be used in structures. We have seen, previously, the “ date” structure as follows:
Struct date
{
int month;
int day;
int year;
}
Just as we have defined the type struct date as in:
struct date birthday
We also define a variable to be a pointer to the structure
Struct date * date pointer and then say
Date pointer = &birthday;
Then, we can go on to access, say the month of the birthday by saying (*date pointer),month. We will now see an example:
program to illustrate structure pointers
main()
{
struct date
{
int month;
int day;
int year;
};
struct date today, *date_pointer;
date_pointer = &today;
date_pointer ->month = 9;
date_pointer ->day = 25;
date_pointer ->year = 1988;
printf(“Today’s date is %d/%d/%d.\n”,date_pointer->month,date_pointer->day,
date_pointer->year %100);
}
OUTPUT:
Today’s date is 9/25/88.
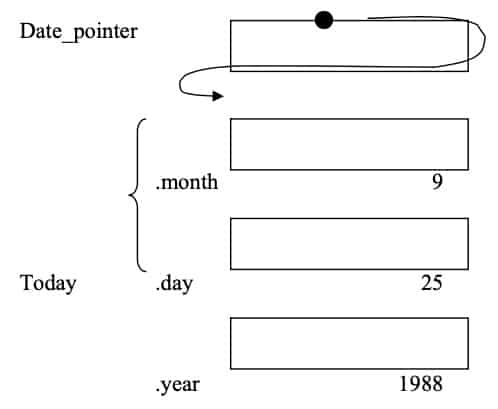
This was about pointers to structures. We can also have pointers in structures. i.e the structure elements themselves can be pointers. Something like:
Struct int pointers
{
int *p1;
int *p2;
};
See the following example.
/* structures containing pointers */
main()
{
struct int_pointers
{
int *p1;
int *p2;
};
struct int_pointers pointers;
int il = 100,i2;
pointers.p1 = &i1;
pointers.p2 = &i2;
*pointers.p2= -97;
printf (“ il = %d, *pointers.p1 = %d\n”, i1, *pointers.p1);
printf (“i2 = %d, *pointers.p2 = %d\n”, i2, *pointers.p2);
}
OUTPUT:
i1 = 100, *pointers.p1 = 100
i2 = -97, *pointers.p2 = -97