How to save output or errors to a file with Linux shell redirection
Standard Input, Standard Output and Standard Error
A running program, or process, needs to read input from somewhere and write output to somewhere. A command run from the shell prompt normally reads its input from the keyboard and sends its output to its terminal window.
A process uses numbered channels called file descriptors to get input and send output. All processes start with at least three file descriptors. Standard input (channel 0) reads input from the keyboard. Standard output (channel 1) sends normal output to the terminal. Standard error (channel 2) sends error messages to the terminal. If a program opens separate connections to other files, it may use higher-numbered file descriptors.
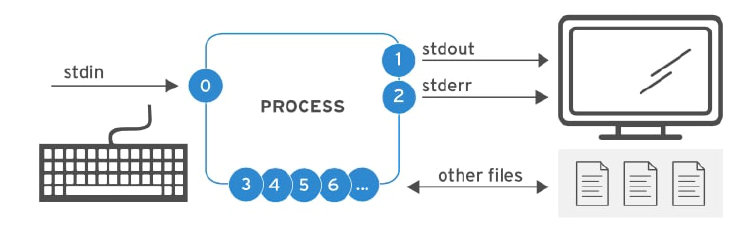
NUMBER | CHANNEL NAME | DESCRIPTION | DEFAULT CONNECTION | USAGE |
---|---|---|---|---|
0 | stdin | Standard input | Keyboard | read only |
1 | stdout | Standard output | Terminal | write only |
2 | stderr | Standard error | Terminal | write only |
3+ | filename | Other files | none | read and/or write |
Redirecting output to a file
I/O redirection changes how the process gets its input or output. Instead of getting input from the keyboard, or sending output and errors to the terminal, the process reads from or writes to files. Redirection lets you save messages to a file that are normally sent to the terminal window. Alternatively, you can use redirection to discard output or errors, so they are not displayed on the terminal or saved.
Redirecting stdout suppresses process output from appearing on the terminal. As seen in the following table, redirecting only stdout does not suppress stderr error messages from displaying on the terminal. If the file does not exist, it will be created. If the file does exist and the redirection is not one that appends to the file, the file’s contents will be overwritten. If you want to discard messages, the special file /dev/null quietly discards channel output redirected to it and is always an empty file.
USAGE | EXPLANATION |
---|---|
> file | redirect stdout to overwrite a file |
» file | redirect stdout to append to a file |
2> file | redirect stderr to overwrite a file |
2> /dev/null | discard stderr error messages by redirecting to /dev/null |
> file 2>&1 | redirect stdout and stderr to overwrite the same file |
» file 2>&1 | redirect stdout and stderr to append to the same file |
> file 2>&1
However, the next sequence does redirection in the opposite order. This redirects standard error to the default place for standard output (the terminal window, so no change) and then redirects only standard output to file.
2>&1 > file
Because of this, some people prefer to use the merging redirection operators:
&>file instead of >file 2>&1
&>>file instead of >>file 2>&1 (in Bash 4)
However, other system administrators and programmers who also use other shells related to bash (known as Bourne-compatible shells) for scripting commands think that the newer merging redirection operators should be avoided, because they are not standardized or implemented in all of those shells and have other limitations.
Examples for Output Redirection
Many routine administration tasks are simplified by using redirection. Use the previous table to assist while considering the following examples:
1. Save a time stamp for later reference.
[user@host ~]$ date > /tmp/saved-timestamp
2. Copy the last 100 lines from a log file to another file.
[user@host ~]$ tail -n 100 /var/log/dmesg > /tmp/last-100-boot-messages
3. Concatenate four files into one.
[user@host ~]$ cat file1 file2 file3 file4 > /tmp/all-four-in-one
4. List the home directory’s hidden and regular file names into a file.
[user@host ~]$ ls -a > /tmp/my-file-names
5. Append output to an existing file.
[user@host ~]$ echo "new line of information" >> /tmp/many-lines-of-information
[user@host ~]$ diff previous-file current-file >> /tmp/tracking-changes-made
6. The next few commands generate error messages because some system directories are inaccessible to normal users. Observe as the error messages are redirected. Redirect errors to a file while viewing normal command output on the terminal.
[user@host ~]$ find /etc -name passwd 2> /tmp/errors
7. Save process output and error messages to separate files.
[user@host ~]$ find /etc -name passwd > /tmp/output 2> /tmp/errors
8. Ignore and discard error messages.
[user@host ~]$ find /etc -name passwd > /tmp/output 2> /dev/null
9. Store output and generated errors together.
[user@host ~]$ find /etc -name passwd &> /tmp/save-both
10. Append output and generated errors to an existing file.
[user@host ~]$ find /etc -name passwd >> /tmp/save-both 2>&1